引入路径
在浏览器环境引入node_modules包时会报错:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> <script type="module"> import { createApp } from 'vue' console.log(createApp()) </script> </body> </html>
|
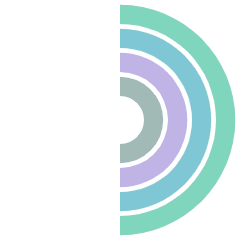
因为在浏览器环境使用ESM引入模块时,只支持解析相对路径或绝对路径
在基于webpack等打包文件的工程中可以这样引入node_modules包,是因为它们基于Nodejs环境
Nodejs底层会为引入路径作判断:如果不是相对或绝对路径,就会在node_modules中寻找模块
但浏览器环境显然是没人做这份活的,只好老老实实通过相对路径引入
1
| import { createApp } from './node_modules/vue/dist/vue.esm-browser.js'
|
importmap
当然这都啥时候了,其实浏览器早已给出了解决方案:importmap
只需要另开一个<script>,在里面写JSON格式的配置即可,它能将引入名映射到一个指定的路径
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> <script type="importmap"> { "imports": { "vue": "./node_modules/vue/dist/vue.esm-browser.js" } } </script> <script type="module"> import { createApp } from 'vue' console.log(createApp()) </script> </body> </html>
|
并发加载
可以结合使用函数模式import和Promise.all
1 2 3 4 5
| <script type="module"> Promise.all([import('vue'), import('vue-router')]).then(res=> { console.log(res) }) </script>
|