反向代理
反向代理(Reverse Proxy)是一种网络通信模式,它充当服务器和客户端之间的中介,将客户端的请求转发到一个或多个后端服务器,并将后端服务器的响应返回给客户端
反向代理的特点如下
- 负载均衡:反向代理可以根据预先定义的算法将请求分发到多个后端服务器,以实现负载均衡,避免某个后端服务器过载,提高整体性能和可用性
- 高可用性:反向代理可以将请求转发到多个后端服务器,以提供冗余和故障转移,如果一个后端服务器出现故障,代理服务器可以将请求转发到其他可用的服务器,从而实现高可用性
- 缓存和性能优化:反向代理可以缓存静态资源或经常访问的动态内容,以减轻后端服务器的负载并提高响应速度,还可以通过压缩、合并和优化资源等技术来优化网络性能。
- 安全性:反向代理可以作为防火墙,过滤恶意请求、检测和阻止攻击,并提供安全认证和访问控制,保护后端服务器免受恶意请求和攻击
- 域名和路径重写:反向代理可以根据特定的规则重写请求的域名和路径,以实现 URL 路由和重定向,能提高系统架构的灵活性和可维护性
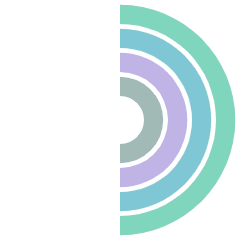
使用
需要先安装http-proxy-middleware
库
1
| pnpm install http-proxy-middleware
|
proxy代理配置项:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| module.exports = { serve: { proxy: { '/api': { target: 'http://localhost:1919', changeOrigin: true, pathRewrite: { '^/api': ' } } } } }
|
端口11451,使用/api
代理http://localhost:1919
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| const http = require('node:http') const url = require('node:url') const fs = require('node:fs')
const { createProxyMiddleware } = require('http-proxy-middleware') const config = require('./my.config.js')
const html = fs.readFileSync('index.html', 'utf-8')
http.createServer((req, res) => {
const { pathname } = url.parse(req.url)
const proxyList = Object.keys(config.serve.proxy)
proxyList.forEach(path => { if(pathname.startsWith(path)) { const proxy = createProxyMiddleware(config.serve.proxy[path]) proxy(req, res) return } })
res.writeHead(200, { 'Content-Type': 'text/html', })
if(pathname === '/') { res.end(html) }
}).listen(11451, () => { console.log('http://localhost:11451') })
|
端口1919,11451端口/api
开头的路由请求通过代理转发到了这里
1 2 3 4 5 6 7 8 9 10 11 12 13
| const http = require('node:http') const url = require('node:url')
http.createServer((req, res) => { const { pathname } = url.parse(req.url) console.log(pathname)
if(pathname === '/test') { res.end('hello api') } }).listen(1919, () => { console.log('http://localhost:1919') })
|
http://localhost:11451/api/test
被成功转发到http://localhost:1919/test
了
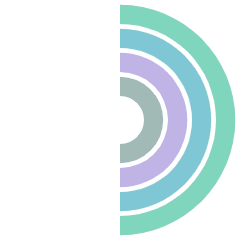